Authentication with the Block-Auth SDK
This guide will get you all set up and ready to use the Block-Auth SDK. We'll cover how to get started using one of our SDK clients and how to make your first SDK request. We'll also look at where to go next to find all the information you need to take full advantage of our powerful REST SDK.
Before you can make requests to the Block-Auth SDK, you will need to grab your SDK keys from your dashboard. You find it under Applications » Settings.
Choose your client
Before making your first request, you need to pick which SDK client you will use. Block-Auth offers clients for JavaScript. In the following example, you can see how to install client.
# Install the Protocol JavaScript SDK
npm install blockauth-sdk-react --save
Connecting using credentials
After picking your preferred client, you are ready to make your first call to the Protocol SDK. Below is an example of how to connect your users with the BlockAuth SDK using React.
We will focus the example over 2 main components FlowBlockAuth
and DropdownProfile
that will help you to connect your users with the BlockAuth SDK.
User actions 1/3: Click on button
Component which starts the sign in/sign up flows <FlowBlockAuth />
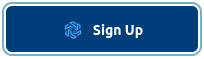
User actions 2/3: Connect with a service
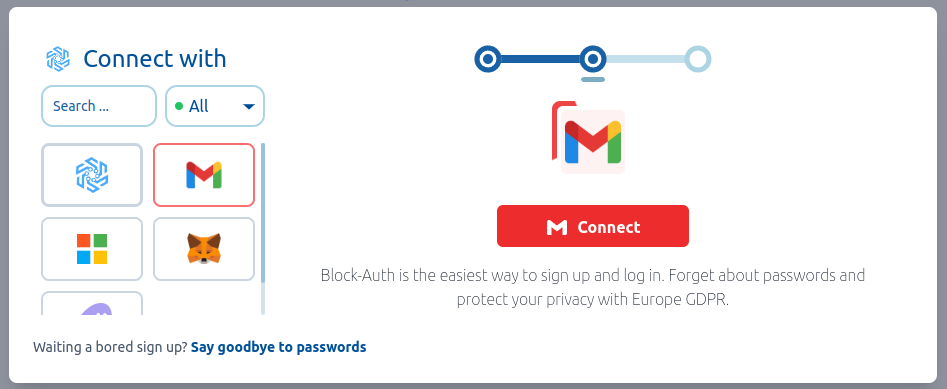
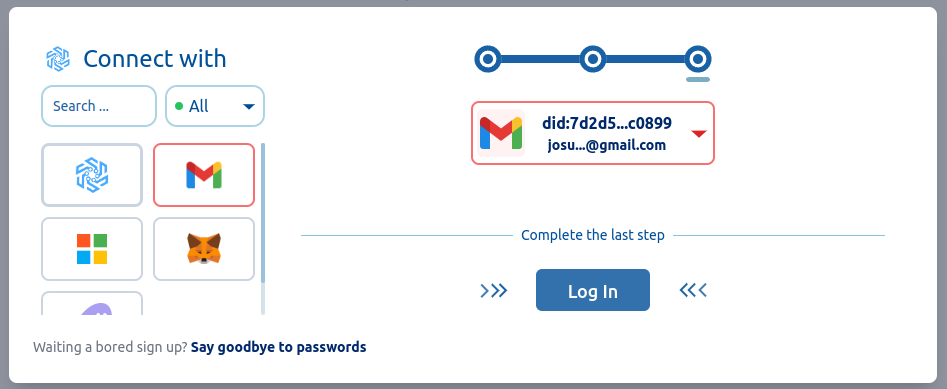
User actions 3/3: Authorize Log In
Component which shows the user profile and allows to log out <DropdownProfile />
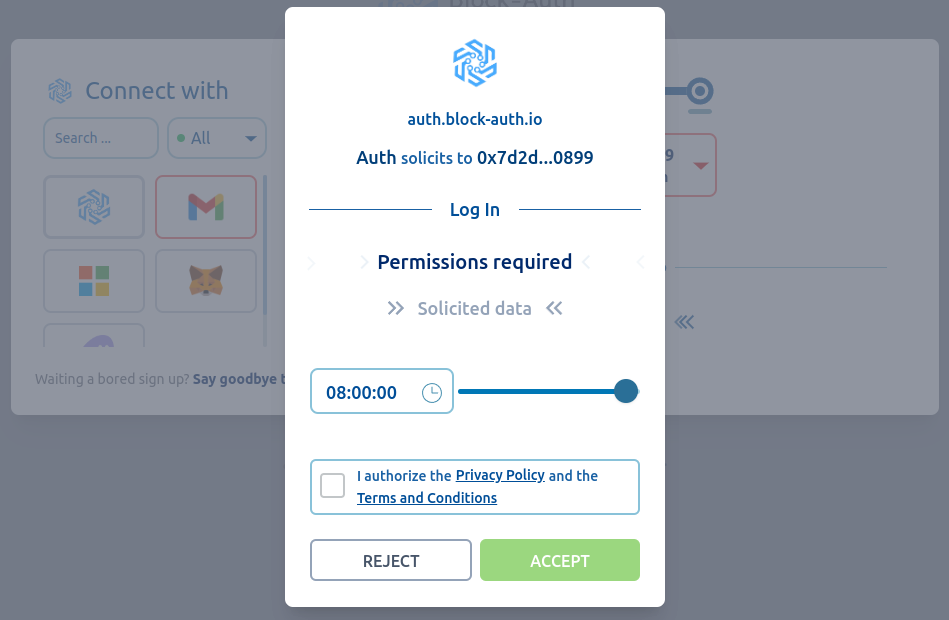
import {
// components
BtnSignin,
BtnGmail,
BtnMetamask,
BtnMicrosoft,
BtnPhantom,
ContentSignin,
ContentSignup,
DropdownProfile,
FlowBlockAuth,
// helpers
accountShort,
cleanUrl,
getIp,
isValidImgUrl,
secsToText,
// stores
useStoreGoogle,
useStoreMetamask,
useStoreMicrosoft,
useStorePhantom,
useStoreModals,
useStoreUI,
useStoreWS,
} from 'blockauth-sdk-react'
import React, { useState } from 'react'
// use your own config
const config = {
api_key: 'your_api_key',
api_secret: 'your_api_secret',
}
// external file for your backend api calls
async function myBackendIsLogguedCall({ address }) {
const apiUrl = 'https://my-backend.com/api/'
// my backend api call must generate a token for the user
const r = await axios.post(`${apiUrl}auth/signin`, { address })
if (r.data.error) return false
localStorage.setItem('auth', JSON.stringify(r.data))
return true
}
export default function App(){
const [isLogged, setIsLogged] = useState(
localStorage.getItem('auth')?.token ? true : false
)
const onLogout = () => {
localStorage.removeItem('auth')
setIsLogged(false)
window.location.reload()
}
const onSuccess = (address) => {
console.log('[front] query api for isSigned: ', address)
const isLogged = await myBackendIsLogguedCall(address)
window.location.reload()
}
return (<>
{(isLogged) && <>
<DropdownProfile
onLogout={onLogout}
apiKey={config.api_key}
apiSecret={config.api_secret}
/>
</>}
{(!isLogged) && <>
<FlowBlockAuth
apiKey={config.api_key}
apiSecret={config.api_secret}
onSuccess={onSuccess}
/>
</>}
</>)
}
What's next?
Great, you're now set up with an SDK client and have made your first request to the SDK. Here are a few links that might be handy as you venture further into the Protocol SDK: